Other Folding Curves
Working with Patterns
We went over how the two Folding Curve functions work in the previous sections, so I won't delve into that again now. What I will say is that you're now free to explore any pattern. In the Levy C curve, you always turned the same direction. In the Dragon Curve, you always alternated directions. But why limit yourself to that? Here, you can specify any set of directions with an array of 1's and 0's.
Folding_Curve(16,0,[-.75,.5],[-.75,.5],[0,1,0,0,1,1])
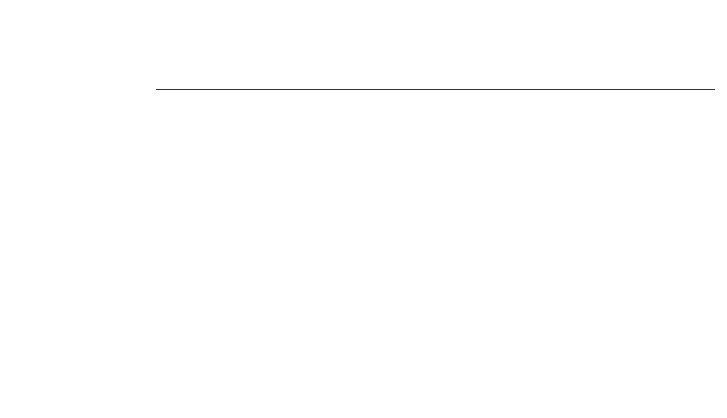

Folding_Curve(15,0,[-1,.75],[-1,.75],[0,1,1,0])
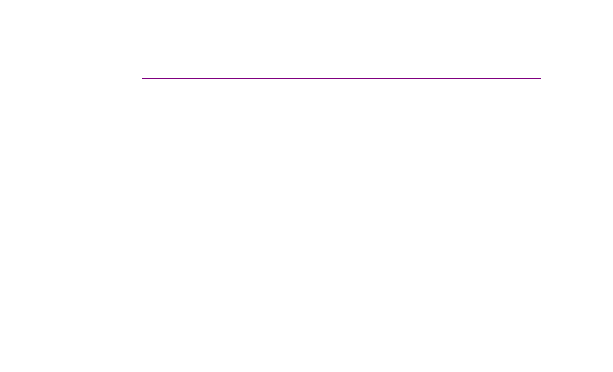

Folding_Curve(15,0,[-1,1],[-1,1],[0,0,1,1])
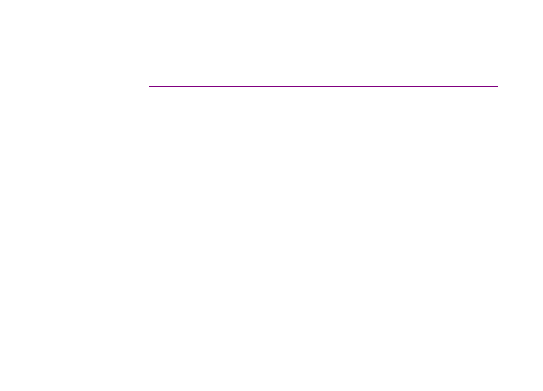
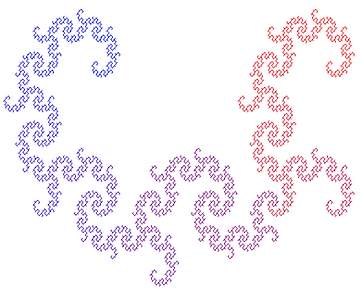
Starting with Four Lines (Tiling)
Folding_Curve_4lines(14,0,[-2.5,2.5],[-2.5,2.5],[1,0,0,1],2)

Folding_Curve_4lines(14,0,[-2.5,2.5],[-2.5,2.5],[1,0,0,1],4)

Folding_Curve_4lines(14,0,[-2.5,2.5],[-2.5,2.5],[0,1,1,0],4)
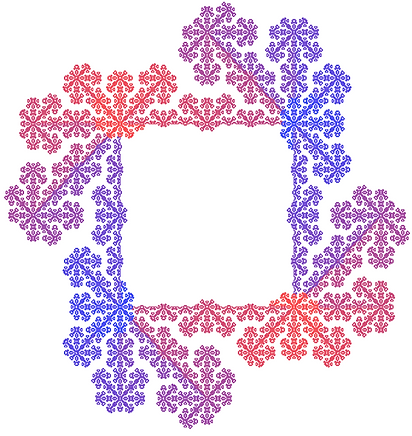
Starting with a Square
In the previous examples, we either used a single line as our starting point, or used four disconnected lines arranged in a square. But what if we actually started with the square? The difference between this and the 4lines version of the code is that we now treat the entire square as an instance of the Folding Curve. When we used four lines, we would follow the folding pattern independently for every line of that square. Now, we cycle the folding pattern throughout the entire square!
The one additional input is the "color" input, which can be 0, 1, or 2. If it's zero, you'll see the red fading into blue color scheme we've been using. If you set it to 1, you'll get a rainbow color scheme. If you set it to 2, you'll get each quarter of the square colored differently.
function Folding_Curve_Square(iterations,makeVideo,xlimVals,ylimVals,pattern,fourColors)
% This function draws a Dragon Curve (or a variant of that curve) with the
% following inputs:
% iterations = int; number of iterations
% makeVideo = 1 or 0; set to 1 to make a video
% xlimVals = [xmin,xmax]; set the x-boundaries for the graph
% ylimVlas = [ymin,ymax]; set the y-boundaries for the graph
% pattern = array of 1's and 0's. For each 0, the pattern will turn
% right. For each 1 the pattern will turn left. When the pattern reaches
% the end, it will repeat. For example, [0,1] will generate the standard
% dragon curve
% fourColors = 0,1,or 2; determines the color of the curve
% 0 = curve is blue fading into red
% 1 = curve is rainbow
% 2 = curve is split into four segments, each a different color
% If making a video, initialize the video object
if makeVideo==1
writerObj = VideoWriter('foldingCurveVideo.mp4','MPEG-4');
writerObj.FrameRate = 1;
writerObj.Quality=100;
open(writerObj)
end
angle=45;
pts=[-.5,-.5;.5,-.5;.5,.5;-.5,.5;-.5,-.5];
len=1;
% Initialize the figure
fig1=figure('Position',[50,50,900,900],'Color',[1 1 1]);
c=[0 0 1];
% Draw the initial square
plot([-0.5 0.5 0.5 -0.5 -.5],[-.5 -.5 .5 .5 -.5],'Color',c,'LineWidth',.75)
hold on; xlim(xlimVals);ylim(ylimVals);axis off
pause(1)
% If making a video, grab a frame
if makeVideo==1
frame = getframe(1);
writeVideo(writerObj, frame)
end
% Repeat for the given number of iterations
for i=1:iterations
disp(i)
cla
len=0.5*len/cosd(angle);
temp=[];
dir=1;
% Repeast for each line segment in the curve
for j=1:size(pts,1)-1
% Grab the two endpoints of the curve
pt1=pts(j,:);
pt2=pts(j+1,:);
% Subtract one endpoint from the other so you're centered on
% zero and can more easily calculate the angle
pt2corr=pt2-pt1;
if 0>=pt2corr(1) && 0>=pt2corr(2)
theta=atand(abs(pt2corr(2)/pt2corr(1)))+180;
elseif 0>=pt2corr(1)
theta=180-atand(abs(pt2corr(2)/pt2corr(1)));
elseif 0>=pt2corr(2)
theta=360-atand(abs(pt2corr(2)/pt2corr(1)));
else
theta=atand(abs(pt2corr(2)/pt2corr(1)));
end
% Determine the new point (the 'midpoint') for your line segment
if pattern(dir)==0 % If turning RIGHT
midpt=[len*cosd(theta-angle)+pt1(1),len*sind(theta-angle)+pt1(2)];
else % If turning LEFT
midpt=[len*cosd(theta+angle)+pt1(1),len*sind(theta+angle)+pt1(2)];
end
% Determine the color based on the current iteration
if fourColors==0
c=[j/size(pts,1) 0 1-j/size(pts,1)];
elseif fourColors==1
tempMap=hsv(size(pts,1));
c=tempMap(j,:);
else
if j>(3/4)*size(pts,1)
c=[1,0,0];
elseif j>(1/2)*size(pts,1)
c=[0,1,0];
elseif j>(1/4)*size(pts,1)
c=[0,0,1];
else
c=[0 0 0];
end
end
% Plot the new line segments
plot([pt1(1),midpt(1),pt2(1)],[pt1(2),midpt(2),pt2(2)],'Color',c,'LineWidth',.75)
hold on
xlim(xlimVals);
ylim(ylimVals);
axis off
% Add the new points to the temp variable
temp=[temp;pt1;midpt];
% Change direction based on the pattern
if dir==length(pattern)
dir=1;
else
dir=dir+1;
end
end
temp=[temp;pts(end,:)];
pts=temp;
% If making a video, grab a frame
if makeVideo==1
frame = getframe(1);
writeVideo(writerObj, frame)
end
pause(1)
end
% If making a video, grab a few frames of the final video and close the
% video object
if makeVideo==1
for i=1:5
frame = getframe(1);
writeVideo(writerObj, frame)
end
close(writerObj)
end
end
Folding_Curve_Square(13,0,[-1.5,1.5],[-1.5,1.5],[0,1,1,0],0)
Folding_Curve_Square(13,0,[-1.5,1.5],[-1.5,1.5],[1,0,0,1],0)
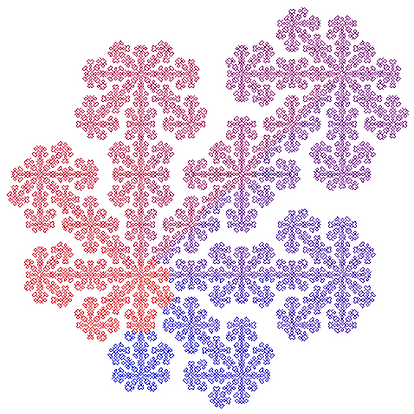

Folding_Curve_Square(14,0,[-1.5,1.5],[-1.5,1.5],[0,0,0,0,1,1,1,1],0)
Folding_Curve_Square(14,0,[-1.5,1.5],[-1.5,1.5],[1,1,0,0],0)
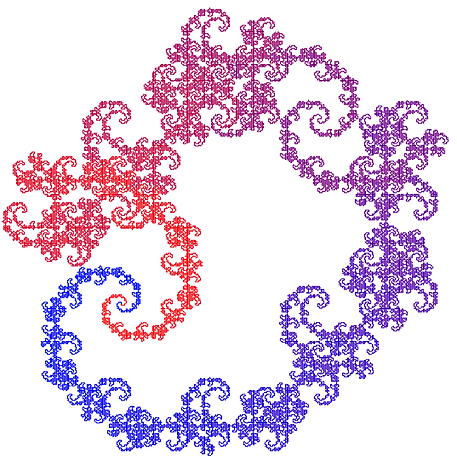
